Performance matters when working with Remixpapa MSW. Whether handling API mocking, streamlining development, or improving testing workflows, the right approach makes a difference. This guide breaks down key features, setup steps, and best practices to help you get the most out of the tool. Avoid common pitfalls, explore advanced capabilities, and fine-tune settings for efficiency. Keep reading to learn how to make Remixpapa MSW work at its best.
Getting Started: Installation and Setup
Setting up Remixpapa MSW correctly helps avoid performance issues and makes integration smoother. Before starting, check system requirements to prevent compatibility problems.
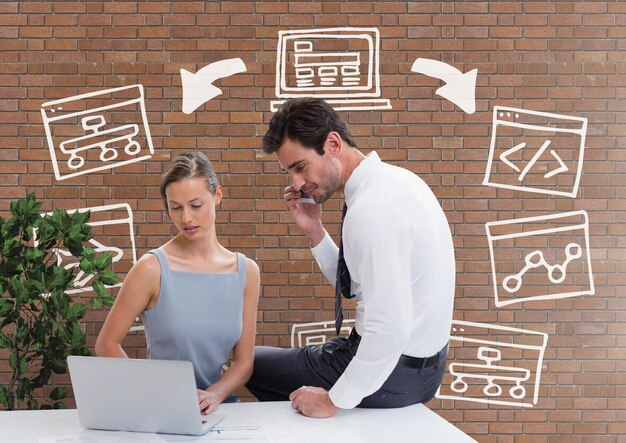
System Requirements
Remixpapa MSW runs efficiently on modern development environments. Ensure the following before installation:
- Node.js (Latest LTS version recommended)
- NPM or Yarn for package management
- A compatible framework (Remix, React, or other JavaScript frameworks)
Keeping dependencies updated improves stability and security.
Step-by-Step Installation Guide
- Install MSW Package
Open a terminal in your project directory and run:npm install msw --save-dev
Or, if using Yarn:yarn add msw --dev
- Set Up the Service Worker
MSW requires a service worker for interception in a browser environment. Generate it with:npx msw init public/
This creates a worker file inside thepublic/
directory. - Create Mock Handlers
Define API request handlers in a new file, such asmocks/handlers.js
:import { rest } from 'msw'; export const handlers = [ rest.get('/api/user', (req, res, ctx) => { return res(ctx.json({ id: 1, name: 'John Doe' })); }), ];
- Configure the Server or Worker
Set up the mock server inmocks/browser.js
for frontend applications:import { setupWorker } from 'msw'; import { handlers } from './handlers'; export const worker = setupWorker(...handlers);
If using MSW for backend testing, configure it inmocks/server.js
:import { setupServer } from 'msw/node'; import { handlers } from './handlers'; export const server = setupServer(...handlers);
- Start the Mocking Process
- In frontend applications, start the worker inside
index.js
orindex.ts
:import { worker } from './mocks/browser'; worker.start();
- For backend or test environments, activate the server in
setupTests.js
:import { server } from './mocks/server'; beforeAll(() => server.listen()); afterEach(() => server.resetHandlers()); afterAll(() => server.close());
- In frontend applications, start the worker inside
Initial Configuration for Efficiency
- Organize Mock Handlers: Group similar requests into separate files to keep the codebase manageable.
- Use Environment Variables: Load mock settings dynamically based on development or testing needs.
- Limit Unnecessary Requests: Disable MSW in production to prevent interference with live APIs.
Once everything is set up, MSW will intercept requests and provide mock responses, allowing faster development and more controlled testing.
Essential Features and How to Use Them
Remixpapa MSW offers a structured way to mock API requests, control responses, and test applications without relying on live servers. Understanding its key functions helps improve efficiency and accuracy in development.
Creating and Managing Mock Handlers
Mock handlers define how MSW intercepts and responds to network requests. These handlers can be customized for different request types.
- Handling GET Requests:
import { rest } from 'msw'; export const handlers = [ rest.get('/api/user', (req, res, ctx) => { return res(ctx.json({ id: 1, name: 'Alice' })); }), ];
This example intercepts requests made to/api/user
and responds with a JSON object. - Handling POST Requests:
rest.post('/api/login', (req, res, ctx) => { return res(ctx.status(200), ctx.json({ message: 'Login successful' })); });
Thectx.status(200)
sets the response status, andctx.json()
sends a structured response. - Dynamic Response Handling:
Requests can be processed based on input parameters.rest.get('/api/user/:id', (req, res, ctx) => { const { id } = req.params; return res(ctx.json({ id, name: `User ${id}` })); });
Here,:id
acts as a variable, making the handler flexible for multiple requests.
Integrating MSW into Your Workflow
- Using MSW in Development
Running MSW in a development environment prevents reliance on external APIs, speeding up debugging. To activate it:import { worker } from './mocks/browser'; worker.start();
This loads handlers and intercepts requests automatically. - Mocking APIs in Tests
When testing, MSW simulates real API behavior, avoiding unpredictable network conditions.import { server } from './mocks/server'; beforeAll(() => server.listen()); afterEach(() => server.resetHandlers()); afterAll(() => server.close());
This setup initializes the mock server before tests run and resets handlers after each test. - Bypassing MSW in Production
MSW should not interfere with live environments. Disabling it when moving to production avoids unnecessary request interception.if (process.env.NODE_ENV === 'development') { worker.start(); }
Utilizing Advanced Capabilities
- Conditional Responses: Modify responses based on query parameters or request headers.
rest.get('/api/products', (req, res, ctx) => { const category = req.url.searchParams.get('category'); return res(ctx.json({ category, products: [] })); });
- Simulating Delays and Errors: Useful for testing how an application handles slow connections or failed requests.
rest.get('/api/data', (req, res, ctx) => { return res(ctx.status(500), ctx.json({ error: 'Server error' })); });
- Logging Requests for Debugging: Helps track intercepted requests during development.
worker.on('request:start', (req) => { console.log('Intercepted:', req.url); });
By understanding and applying these features, Remixpapa MSW can be used effectively to create reliable mock environments, improve testing accuracy, and maintain smooth development workflows.
Optimizing Remixpapa MSW for Maximum Performance
Setting up Remixpapa MSW is only the first step. To keep it running efficiently, adjustments can be made to reduce overhead, speed up development, and avoid unnecessary resource usage.
Best Practices for Efficiency
- Limit Unused Handlers
Large numbers of mock handlers can slow response times. Removing handlers that are no longer needed keeps things running smoothly. - Use Specific Request Matching
Instead of catching broad request patterns, define precise endpoints. This prevents unexpected behavior and reduces processing time.rest.get('/api/user/:id', (req, res, ctx) => { return res(ctx.json({ id: req.params.id, name: 'User' })); });
- Cache Mock Responses
If mock responses remain unchanged, caching them locally reduces processing load.const cachedResponse = { id: 1, name: 'Cached User' }; rest.get('/api/user', (req, res, ctx) => { return res(ctx.json(cachedResponse)); });
Resource Optimization and Advanced Settings
- Reduce Logging in Production
Excessive console logging can slow down applications. Logging should be disabled outside of development.if (process.env.NODE_ENV === 'development') { worker.on('request:start', (req) => { console.log('Intercepted:', req.url); }); }
- Simulate Network Conditions for Testing
Adding artificial delays to API responses helps evaluate how an application handles slow connections.rest.get('/api/data', (req, res, ctx) => { return res(ctx.delay(2000), ctx.json({ message: 'Delayed response' })); });
- Optimize Test Execution
Running unnecessary mocks during tests increases execution time. Use only the required handlers for specific test cases.test('fetches user data', async () => { server.use( rest.get('/api/user', (req, res, ctx) => { return res(ctx.json({ id: 2, name: 'Test User' })); }) ); // Test logic here });
Regular Maintenance and Updates
- Keep Dependencies Updated
Running outdated versions may cause compatibility issues. Update dependencies regularly using:npm update msw
- Clean Up Old Mock Data
Over time, mock data files can grow unnecessarily large. Removing outdated mock responses keeps files manageable. - Monitor Performance
If response times increase, reviewing active handlers and logging activity can help identify bottlenecks.
Making these adjustments improves efficiency, keeps tests reliable, and reduces unexpected issues during development.
Troubleshooting and Common Challenges
Even with proper setup and configuration, issues can arise when using Remixpapa MSW. Recognizing common problems early can save time and help maintain a smooth development process.
Fixing File Upload and Rendering Issues
- File Upload Errors:
When testing file upload functionality, MSW might fail to mock file-related responses correctly. Ensure that the mock server handles the multipart form data appropriately. For instance:rest.post('/api/upload', (req, res, ctx) => { return res(ctx.status(200), ctx.json({ message: 'File uploaded successfully' })); });
If the response is delayed, usectx.delay()
to simulate network lag, helping you test edge cases in the UI. - Rendering Delays in React:
If your React components seem to delay while awaiting mock responses, it could be an issue with how the MSW worker is initialized or the mock data is being processed. Ensure that mock responses are set up before the components mount. AdjustinguseEffect
or other component lifecycle methods may help.
Debugging Collaboration and Testing Problems
- Syncing with Multiple Team Members:
In collaborative development environments, inconsistent mock configurations between team members can lead to different results. To resolve this:- Make sure everyone uses the same version of MSW and related dependencies.
- Store mock definitions in version-controlled files (e.g.,
mocks/handlers.js
). - Standardize how mocks are set up in tests to avoid conflicts.
- Test Failures Due to Mocking Mismatches:
Sometimes, tests might fail because mock handlers don’t match the requests being made by the application. This can happen if the URL or method (GET, POST, etc.) isn’t properly configured in the mock. Double-check that the request paths and parameters in the mock handler exactly match those made by the application.
Ensuring Seamless Frontend-Backend Interaction
- Discrepancies Between Mock and Real APIs:
During development, it’s easy to create mocks that differ slightly from the real API. This can cause unexpected issues when switching to the production environment. To minimize such problems:- Keep mock responses as close as possible to real API structures.
- Test the app with real backend endpoints periodically to spot discrepancies early.
- Mocking Dynamic Responses:
If your application depends on changing data (e.g., user authentication tokens), ensure that your mock handlers return data dynamically instead of using hardcoded responses. For example, simulate a token validation process in the mock:rest.post('/api/login', (req, res, ctx) => { const { username, password } = req.body; if (username === 'admin' && password === 'password123') { return res(ctx.status(200), ctx.json({ token: '123456' })); } return res(ctx.status(401), ctx.json({ message: 'Invalid credentials' })); });
Resolving Server-Side Testing Issues
- Server Setup and Reset:
Sometimes, mock servers may not reset as expected between tests, causing stale mock responses. Useserver.resetHandlers()
after each test to clear outdated mocks and avoid conflicts.afterEach(() => server.resetHandlers());
- Server Initialization in Test Suites:
Incorrect server initialization order can lead to failures in intercepting network requests during tests. Make sure to callserver.listen()
before tests run and clean up afterward:beforeAll(() => server.listen()); afterAll(() => server.close());
By resolving these common issues, you can keep Remixpapa MSW running smoothly and focus on more important tasks like feature development and testing.
Final Thoughts and Key Takeaways
After setting up and optimizing Remixpapa MSW, it becomes an invaluable tool for improving your development and testing workflows. Whether you are mocking API requests or simulating network conditions, this tool can drastically reduce dependencies on external services and streamline your entire process.
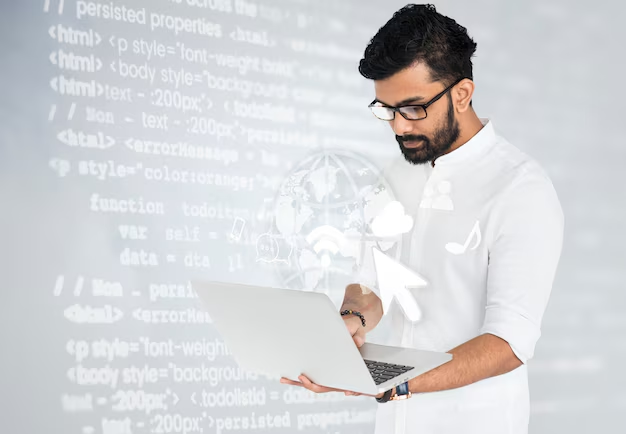
By following best practices, such as limiting unnecessary handlers, regularly updating the software, and ensuring correct configurations, you’ll be able to work with Remixpapa MSW more efficiently. Managing mock data carefully and using dynamic responses can help ensure that your development environment reflects real-world scenarios.
Troubleshooting common issues such as file upload errors, rendering delays, and test failures will save time and allow you to focus on more critical aspects of your project.
In the end, Remixpapa MSW isn’t just a tool for mocking requests—it’s a key component of a smooth, efficient, and high-performing development pipeline.
Conclusion
Remixpapa MSW offers a powerful approach to handling mock APIs in web development. By following the setup and optimization tips provided, developers can maximize performance and avoid common pitfalls. Proper configuration, efficient resource management, and consistent troubleshooting will help keep projects running smoothly. This tool is an asset for building reliable, scalable applications while minimizing dependencies on real external services during testing and development.